**A Childlike Perspective of the Verse Programming Language**
Hoan Nguyen @Mushogenshin Media
As Speaker Proposal for Unreal Fest Orlando 2025
!!! UPDATE
See my [Unreal Fest Bali's version](/bali-2025) of this essay.
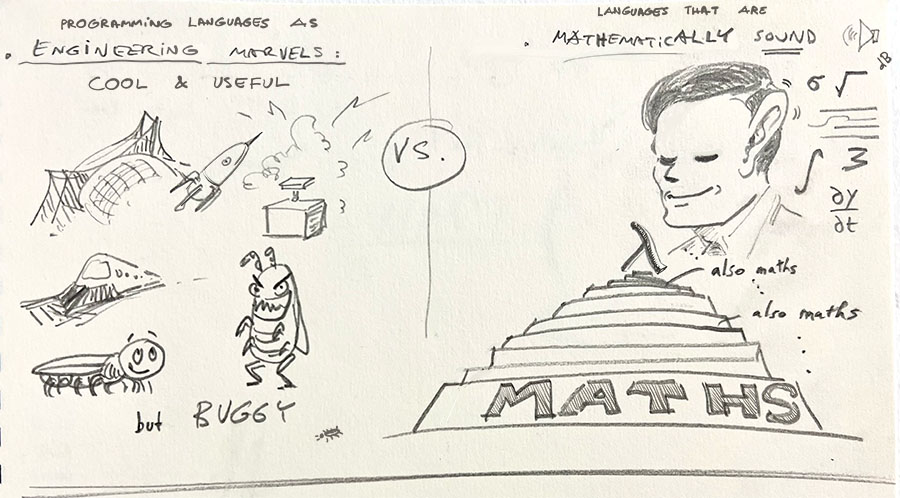
!!! WARNING
This essay is a work in progress.
In this essay, I aim to present a fresh perspective on the Verse
programming language, a creation of Epic Games [unveiled at GDC
2023](https://youtu.be/5prkKOIilJg). By "fresh," I mean an approach that
diverges from the conventional focus on syntax and semantics typically found in
technical literature. Instead, I will illuminate the language's inception, its
foundational principles, its underlying motivations, and its potential, all
through the lens of a non-programmer. My aspiration is that, upon completing
this essay, readers will grasp the distinctiveness of Verse and recognize its
value, even if they are not professional programmers.
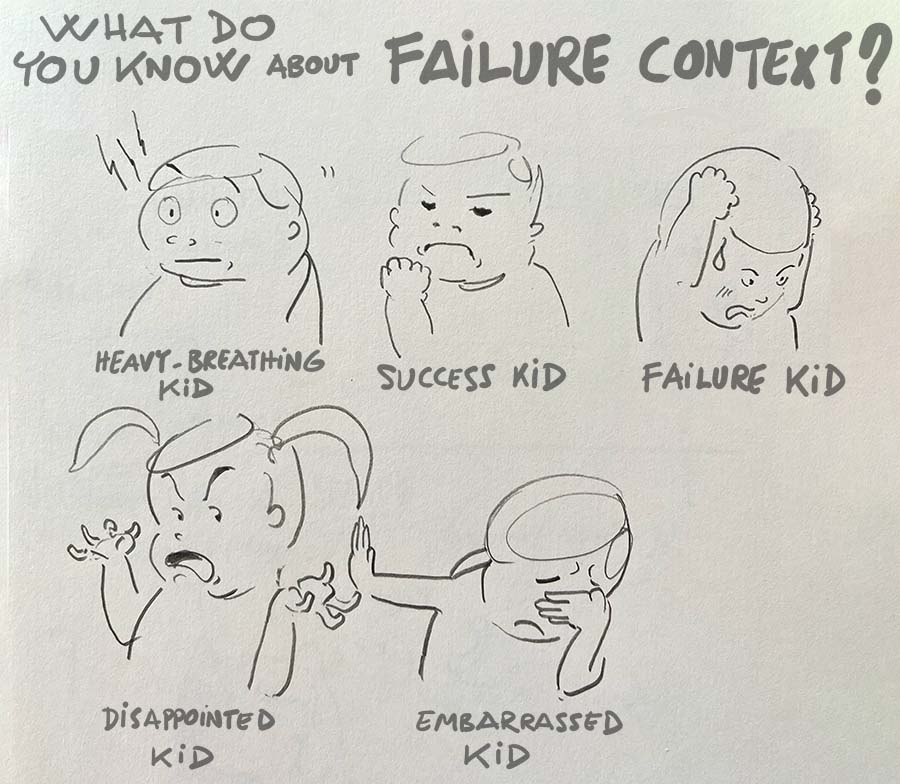
Don't Do These In Verse
=======================
It may seem peculiar to discuss the missteps of programming with Verse before
delving into its essence. However, given that my approach is inspired by a
childlike perspective, it is fitting to begin with a lighthearted exploration of
what to avoid. This mirrors the unfortunate reality where children are often
instructed on prohibitions before comprehending the underlying rationale.
Additionally, it serves as a subtle parody of how our brains are often convinced
that they can learn a thing or two from anything presented in a listicle format.
We'll ramp up to more complex topics in due course, for now, these are the
things to avoid when programming in Verse.
Returning Option Type Instead of Using `decides` Effect in Getters
------------------------------------------------------------------
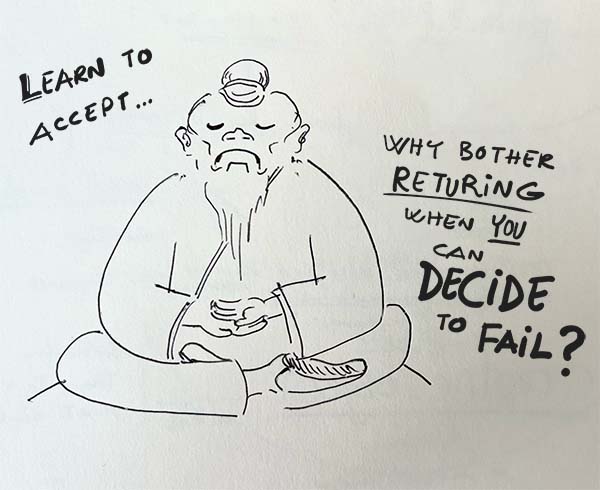
One of the essential and highly beneficial concepts in Verse is the idea of
failure context. With some
[exceptions](https://dev.epicgames.com/documentation/en-us/uefn/verse-code-style-guide-in-unreal-editor-for-fortnite#42getxfunctionsshouldbe),
by adding `decides` and `transacts` specifiers to your getter function, you can
ensure that it fails early if it encounters any issues, rather than returning an
optional result that the user must handle with additional checks. If this
concept seems confusing, especially if you're coming from other mainstream
languages, that's expected. Logic programming and functional programming, which
Verse embraces, will require us to rewire our brain to adapt to this new way of
thinking.
Underutilizing Interfaces for Composition Instead of Inheritance
----------------------------------------------------------------
!!!
A heads-up for non-programmers: in programming, an "interface" is often
considered an advanced topic, distinct from the user interface you see on your
computer screen. Interfaces are typically used to define a set of methods that a
class must implement. They are a powerful tool for structuring your code because
they avoid implementation details and promote composition over inheritance. If
you're familiar with ECS (Entity-Component-System) architecture, commonly used
in game engines, you'll recognize that it's all about composition.
In the diagram below, some fundamental interfaces in Verse are illustrated,
typically represented as adjectives. For example, an `event` is both
`signalable` and `awaitable`, while `listenable` is both `awaitable` and
`subscribable`. In Verse, as in other languages that support interfaces,
interfaces should be used to avoid deeply nested inheritance. Relying solely on
inheritance can make your code inflexible and difficult to maintain over time.
**************************************************************
* listenable
* .-----------------.
* | +
* | awaitable -+----- event
* | ^ | |
* | \ matches |
* | \ with |
* | |\ |
* | | v |
* | | signalable
* | | ^
* | | /
* | |/matches
* | / with
* | v
* | subscribable |
* | ◍ |
* | | |
* | | |
* '-----------------'
* |
* |cancelable
**************************************************************
If you're a Rust programmer, you might be familiar with the concept of
**traits**, which are akin to interfaces in Verse. Like Rust, a class in Verse
can implement multiple interfaces. However, unlike Rust and other languages,
Verse allows you to declare inheritance from a superclass and specify multiple
interfaces simultaneously.
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ python
# This is the definition of the `tag_component` class in Verse.
# It inherits from the `component` class and implements the `tag_view` interface.
tag_component := class(component, tag_view):
# Implementation details...
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
This demonstrates the expressiveness that Verse offers.
Subscription Pitfalls
---------------------
In many "[Build A
Game](https://dev.epicgames.com/documentation/en-us/uefn/build-a-game-in-unreal-editor-for-fortnite)"
UEFN-Verse basic examples, you may see numerous `Subscribe` calls. While
`Subscribe` is an integral part of the event binding process—a common pattern in
game programming and essential when using Fortnite creative devices—it is not
always the optimal way to handle time-flow.
!!!
For those unfamiliar with the concept, event binding is similar to
the "observer pattern" in programming. This means that one part of your program
can announce changes, and other parts can listen for these changes and react
immediately. It's like how a radio transmitter sends signals that receivers
pick up. This concept is also used in other systems like Qt's "signals and
slots," Go's "message passing," and Unreal Engine's "event dispatcher."
Time-flow control requires more than just the subscription system. Imagine the
"Race against a Volcano" scenario discussed by Conan Reis in this
[talk](https://youtu.be/B3WiSgKXsrg?&t=957). Theoretically, you could create a
"`PompeiiForever`" event that `Signal()`s when the volcano erupts. However, it
would be rather silly for every object in the scene to `Subscribe()` to such a
catastrophe, wouldn't it? But it's not only about silliness; the flexibility of
event binding — where the sender doesn't care what the receiver(s) do with the
messages — can become a headache when you need to understand the cause-effect
relationship, and its sender-receiver model can prove limited when you need to
orchestrate complex, time-dependent behaviors that mimic real-life operations.
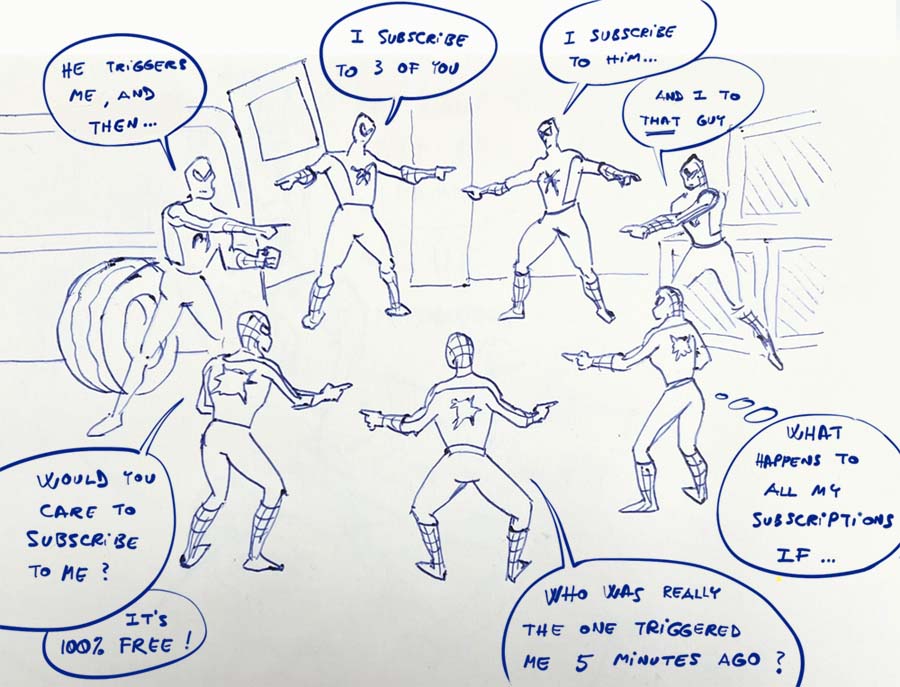
Besides `Await`, Verse offers elegant and robust constructs for expressing
concurrency logic, such as [`sync` and
`race`](https://dev.epicgames.com/documentation/en-us/uefn/concurrency-in-verse)
and some more. By incorporating these new approaches to concurrency programming,
you can define how coroutines interact with each other, such as waiting for one
another or being canceled.
Not Using Extension Method for Single-Parameter Functions
---------------------------------------------------------
TODO
Assuming Async as Rare Cases
----------------------------
!!! WARNING
Async programming may seem like a mind-blowing concept if you fall into one or
more of these categories:
- The last programming course you took was Pascal in high school.
- You have never done web programming.
- Your best coding experience is with MEL or Python 2 in Maya.
- You have never used async loading in Unreal Engine.
Unlike Python or other languages where async is an afterthought, async is woven
deep into the very fibers of Verse. It's not just a feature; it's a fundamental
aspect of the language.
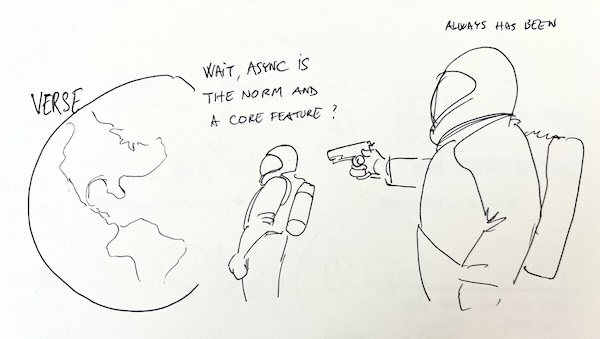
In Verse, you indicate async functions with the `suspends` specifier, making it
clear that the function does not return immediately and takes some time to
complete its work.
Not Limiting Scopes Enough
--------------------------
TODO: `` and ``
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
What Verse Can Do For You
=========================
Having navigated the initial prohibitions, we now turn our attention to a
practical and immediate exploration of Verse. This approach mirrors the natural
inclination of children, who are drawn to toys they can readily manipulate and
engage with, rather than those that demand advanced knowledge or skills to
appreciate their theoretical potential. By focusing on the tangible and
pragmatic aspects of Verse, we can better understand its immediate applications
and benefits, setting aside, for the moment, its more abstract
possibilities.
What 200+ Creative Devices Can't Already Do
-------------------------------------------
In [Creative
1.0](https://dev.epicgames.com/community/fortnite/getting-started/creative?lang=en-US),
you can already build a lot of interesting experiences with fairly complex
gameplay using only creative devices and without touching any code. But Verse
allows your imagination to go even further.
> "Programming is inherent to game design"
> -- On ["Why Verse and Creative?"](https://dev.epicgames.com/documentation/en-us/uefn/verse-language-get-started-in-unreal-editor-for-fortnite#whyverseandcreative?)
A first mental image of what you can do with Verse is to think of it as a hub, a
place to connect all or most of your creative devices. This allows data to flow
between them, and also makes binding functions and events more manageable.
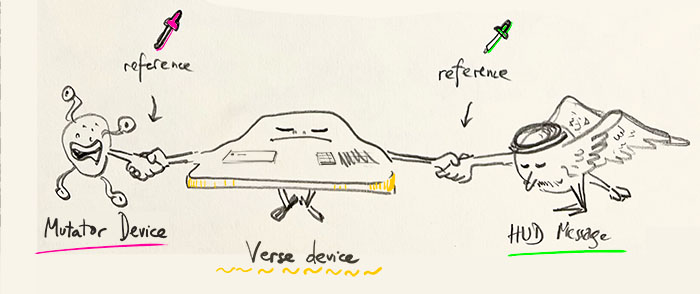
But that's not all. Verse enables you to go above and beyond the pre-defined
behaviors of creative devices and devise your own custom logic. This is where
the real fun begins: like when someone cleverly transformed a [chair
device](https://dev.epicgames.com/documentation/en-us/fortnite-creative/using-chair-devices-in-fortnite-creative)
into a flyable mount with a dragon flapping its wings, simply by using the
[`creative_object.MoveTo()`](https://dev.epicgames.com/documentation/en-us/uefn/verse-api/fortnitedotcom/devices/creative_object/moveto-1)
method and attaching to it a custom `creative_prop` with an animated skeletal mesh.
Unprecedented Concurrency Support: Dedicated Idioms and Expressions
-------------------------------------------------------------------
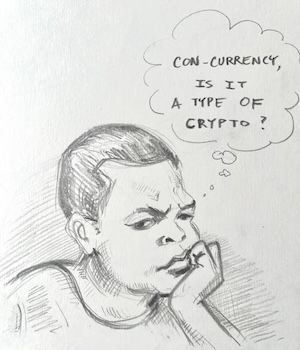
As one of the newest programming languages, Verse learns from the mistakes of
its predecessors, incorporates the best thinking models, and offers a fresh
perspective on concurrency. While it boasts many great features, concurrency is
considered the "most evolved and stable" aspect of the language.
Traditionally, game programming requires you to think about your implementation
in terms of ticks, or updates per frame — changes that occur in a "single,
instantaneous moment of time." Ubiquitous as it is, this approach is
counter-intuitive because it doesn't align with how the human mind perceives
time. To overcome this long-standing challenge, Verse introduces a rich set of
concurrency expressions. Just as natural languages develop distinct vocabularies
and idioms to help people express various concepts effectively, Verse's
concurrency expressions enable you to describe your program's behavior in terms
of the "passage of time," which is more intuitive and natural for human
thinking.
!!!
Remember all the times you are presented with conditions in the form of "event A
**or** event B", such as:
- "The mobile data plan offers unlimited data for 30 days or up to 10 GB of high-speed data, whichever comes first."
- "The software license is valid for 1 year or 5,000 activations, whichever comes first."
- "The rental agreement ends either after 12 months or 30 days after the tenant gives notice, whichever comes first."
Those time logic scenarios are very common in real life and also in game development.
Frequently required in implementations, these time-based conditions can be
challenging to handle cleanly without native language support.
**********************************************************************************
* .--------------.
* | complimentary |
* | automobile |
* | maintenance | .---------.
* | service: race: | | product |
* +---o-----*----+ | warranty: | .----------.
* | | | race: | | health |
* | | +--o---*--+ | insurance: |
* | | 3 | | | race: | .----------.
* | | years | | +--o---*---+ | gym |
* | | | | 2 | | | membership:|
* | | | | years | | | race: | .-----------.
* 30,000 | | | | | +--o---*---+ | promotional |
* miles | 20,000 | | | | | | | offer: |
* | | hours | | $10,000 | 12 | | | race: |
* | | of | | medical | months | | 180 +---o---*---+
* v | usage | | expenses | | | days | |
* | v | | | 50 | | | | until
* | | v | visits | | first | | end
* | | | v | 100 | | of
* | | | | customers | | month
* | | | | v |
* | | | | |
* v v v v v
* ___ ___ ___ ___ ___
*
*
* ⤷ Welcome to the races! ⤶
**********************************************************************************
Above are examples of `OR` time logic; examples of `AND` time logic are also easy to find:
- "I'm only leaving the town once my two puppies reach two years old and my cat's chronic motion sickness is cured."
- "The motorcycle won't start unless you turn the key, hold the brake, put up the kickstand, and press the ignition button for at least 2 seconds."
With Verse's powerful capabilities, you can express time logic in a more natural
way, giving you fine control over both *setup* and *teardown*. This allows you to
manage not only how processes should start but also how they should be canceled
and cleaned up.
!!! Tip: Analogy of Tick to CPU Word
In the same vein that John Backus, in his famous 1977 lecture, criticized the
"von Neumann bottleneck" for its word-at-a-time computation (where "word" refers
to a fixed-sized piece of data that the CPU processes in a single operation) and
tiny state changes, and demonstrated how functional programming helps us think
in terms of conceptual units, e.g. actions and operations, traditional game
programming forces us to think in terms of ticks. In contrast, Verse allows us
to think in terms of stages and time-flow — also conceptual units.
In both cases, the benefit of **not** thinking in the same terms as the
constraints related to computer machinery is that it allows us to reason
about our programs in a more abstract and high-level way.
Immediate-Mode UI Code Meets Unreal's UMG
-----------------------------------------
Simulation Updates without Fretting
-----------------------------------
Procedurally Created Keyframed Movement
---------------------------------------
More Proceduralism: Materials, VFXs, Meshes
-------------------------------------------
Harnessing the Full Potential of Scene Graph
--------------------------------------------
The announcement of Scene Graph support, initially as a beta in UEFN and later
officially in Unreal Engine, is likely to excite those familiar with its
capabilities.
!!!
In computer algorithms and game engines, a scene graph allows for
easy traversal and manipulation of the scene hierarchy, simplifying organization
and management.
Understanding that `prefab` is also `entity` makes the new Scene
Graph straightforward, as it consists solely of entities and components, and
nothing else.
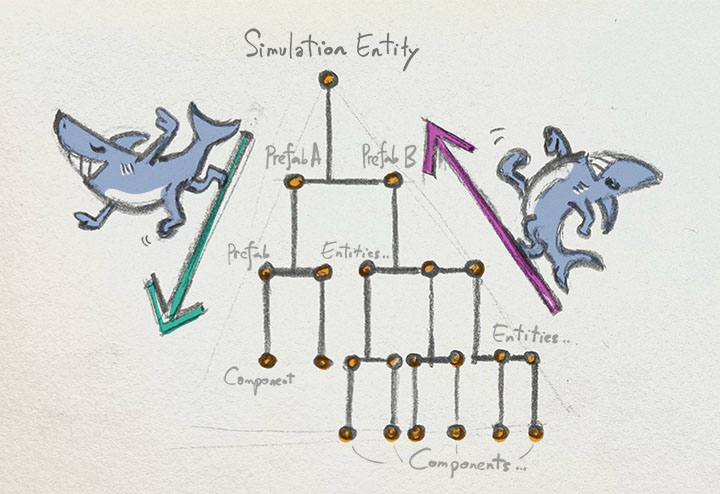
### Components on Roids
!!! WARNING: Not Your Old Components
Some developers who have become proficient with Unreal Engine's traditional
system of actor, child actor, and component may find the introduction of the
Scene Graph confusing and redundant. It's important to understand that, despite
using the same terminology "component," the old and new systems are very
different. The new Scene Graph foundation is built entirely in Verse, and what
sets Scene Graph components apart is that they can contain Verse gameplay logic
on their own. These Verse code components can also be attached to other Scene
Graph entities (or if you wish, actors), making them versatile and powerful.
Below is an example of a rotating component that can be attached to any Scene
Graph entity and conditionally make it rotate. This works by accesses its parent
entity's transform component and if found mutates its local rotation Z at a
constant speed modifiable in editor.
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ python
rotating_component := class(component):
# A custom variable you can expose to the editor
@editable
var Speed:float = 10.0
var MaybeComp: ?transform_component = false
OnBeginSimulation():void =
(super:)OnBeginSimulation()
if (Found := Entity.GetComponent[transform_component]):
set MaybeComp = option{Found}
OnSimulate():void =
loop:
if (Comp := MaybeComp?):
Sleep(0.0)
var LocalTransform: transform := Comp.LocalTransform
set LocalTransform.Rotation = LocalTransform.Rotation.ApplyLocalRotationZ(Speed)
Comp.SetLocalTransform(LocalTransform)
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
The example above is modest and trivial, but it demonstrates the capabilities of
Verse code component. It is designed to revolutionize the way you dynamically
change materials, meshes, animations, and VFX. While you can already achieve a
lot with C++ and Blueprints, Scene Graph and Verse offer a more flexible and
reusable approach, reducing the amount of boilerplate code required, provided
you know how to [structure your
tree](https://dev.epicgames.com/documentation/en-us/uefn/creating-your-own-verse-component-in-unreal-editor-for-fortnite#queryingentitiesandcomponentswithverse).
Transacts and Rollbacks
-----------------------
Optionals and Unwrapping
------------------------
Worry-Free Memory Management
----------------------------
### `Dispose` and `Drop`
First-Class Functions, No, Really
---------------------------------
In Verse, functions are given such a special status that you don't even need a
keyword like `def` or `fn` to declare them.
You also call async functions just like any other function, as long as you're in
a context that allows it.

TODO: functions as arguments, functions as return values, functions as variables
Abstracted Away Recursion
-------------------------
Easy Filtering
--------------
Free, Invaluable, Design Lessons
--------------------------------
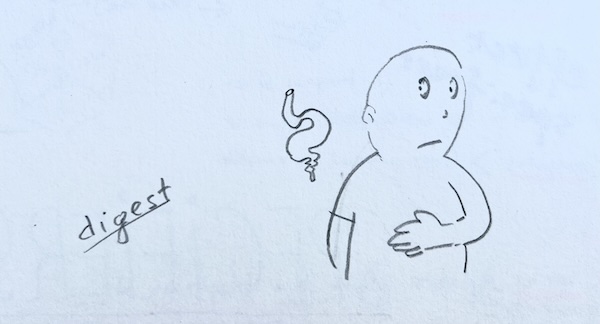
!!! Tip
Just like old-school electrical or mechanical engineers who pore over product
manuals to understand configurations and usages, you can learn a lot about Verse
and also about code design by examining the digest files.
While the endeavor of studying these files may seem boring, they provide a
high-level overview of a modern language that has been carefully thought out,
offering invaluable insights into how various APIs can be organized and defined.
Though you won't see the inner workings, the knowledge gained from these digests
is rewarding and provides illuminating lessons for code design as a whole, not
just for writing code in Verse.
*********************************************************************************
* (Scene Graph) extension
* .-----------. ╔════════════▶ - GetPlayspace()
* | entity ⏣ | ║
* '-----------' ║ ╔═════════▶ - GetTags()
* ║ extension ║ ║
* ║ ║ ║
* - FindCreativeObjectsWithTag() ║ ║ .------------.
* ║ ║ ║ | positional |
* ▼ returns ║ ║ '------+-----'
* .--+-------------------------. ^
* | ⏣ ⏣ | | - GetTransform()
* | creative_object_interface o---------'
* .-------->+ | implements
* | '--------------------+-------'
* | ^
* | .---------------. |
* | | creative_object o-----'
* | '-------*-------' implements
* | .
* | implements |
* | | - TeleportTo()
* .----o----------. | - MoveTo()
*| creative_device | | .------+-----.
* '---------------' | | disposable |
* + '------+-----'
* .--------' '--------. ^
* | | | - Dispose()
* ^ ^ .-------o-------.
* | | | invalidatable |
* inherits | inherits | '-------+-------'
* | | ^
* .---------+-----------. .----+--------. | - IsValid()
*| creative_device_base | | creative_prop o------------'
* '---------------------' '-------------' implements
* ▲ ▲
* ╏ ╏
* ╏ spawns ╏ spawns
* ╏ into ╏ into
* .--◌------------------. .----◌--------------.
* | creative_device_asset | | creative_prop_asset |
* '---------------------' '-------------------'
*********************************************************************************
Demonstrated above is a great example of a loosely coupled design for
`creative_object`, `creative_object_interface`, `creative_device_base`,
`creative_prop`, and also how they are related to Scene Graph and tags.
!!! Tip
By examining the digests, you can quickly see how compact Core Verse truly is,
which is a clear advantage for its future as an open standard.
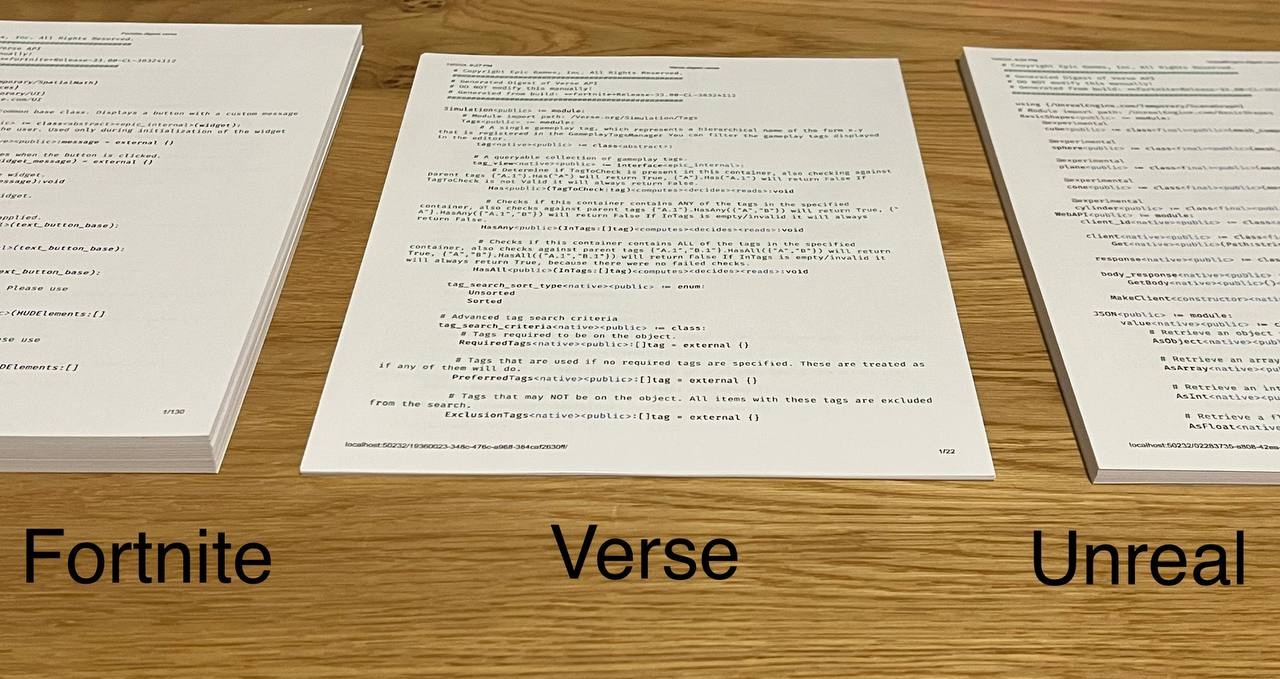
Dry as they may seem, these invaluable, no-cost lessons are right at your
fingertips. They represent the pinnacle of programming knowledge, distilled from
decades of battle-tested experience. This encapsulates what Verse can offer you.
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
Why Verse Is Not Just Another New Language
==========================================
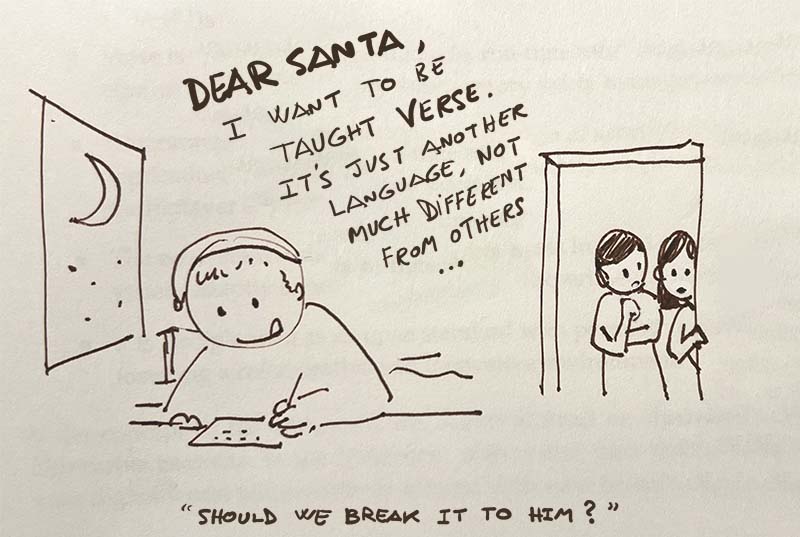
!!! ERROR
A common tendency in many Verse tutorials found online that may potentially
hinder the adoption of Verse is the inclination to teach it by first going over
all features of imperative programming in Verse and with Verse. This approach
obscures where Verse shines the most, if not completely undermining its unique
strengths.
Instead, a more logical approach would be to communicate Verse's distinctive and
innovative programming concepts, highlighting what sets it apart, what
additional capabilities users can achieve with it, and most significantly, what
cognitive burdens it can liberate them from, compared to languages like C#, C++,
Python, JavaScript, Lua, etc.
Same Mathematical Integrity, under the Ivory Tower
--------------------------------------------------
### Purity, Side Effects, and Usefulness
### The Two Meanings of Variables
### Choices
### `where` clauses
### Generators and Other Lazinesses
Type Safety and Type Casting
----------------------------
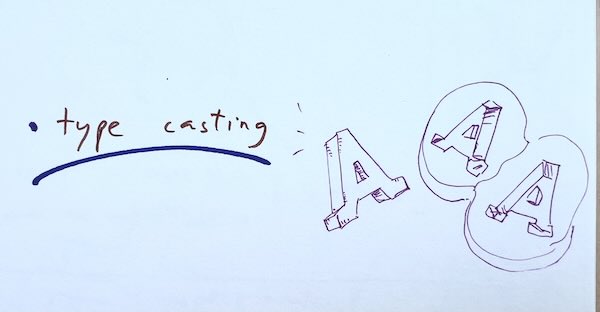
Gameplay Tags
-------------
Module System
-------------
Shared 3D Simulation
--------------------
The term Metaverse has, regrettably, garnered a somewhat tarnished reputation.
This is understandable, given the recent limited approaches and poor attempts,
not to mention the unfortunate conflations with cryptos, NFTs, VR headsets, or
static app stores. However, it is refreshing to realize that the grand vision of
a "shared 3D simulation" as originally conceived in Metaverse literature has yet
to be fully realized. Verse, methodically built from the ground up, is poised to
address the technical challenges inherent in this vision. This represents our
best opportunity to bring this concept to fruition, thanks to several key
principles:
- Verse is a "compile-time verifiable, run-time safe" language, but also eliminates the need for manual memory safety management.
- Programmers are relieved from the burden of specifying network replications, allowing for more streamlined development of multiplayer experiences.
- The ecosystem is designed with robustness in mind under a coherent vision, adeptly handling deprecated or experimental features.
- It is also planned as an open standard with permissive licenses, fostering a collaborative and innovative environment.
If the concept of the Metaverse still seems abstract or elusive, consider this
illustrative example: In the Metaverse, objects and their defined attributes in
your digital world will seamlessly interact with your friend's digital world.
For instance, if you ride a wrecking ball to a planet with much lower gravity,
you'll bounce off it like a beach ball.
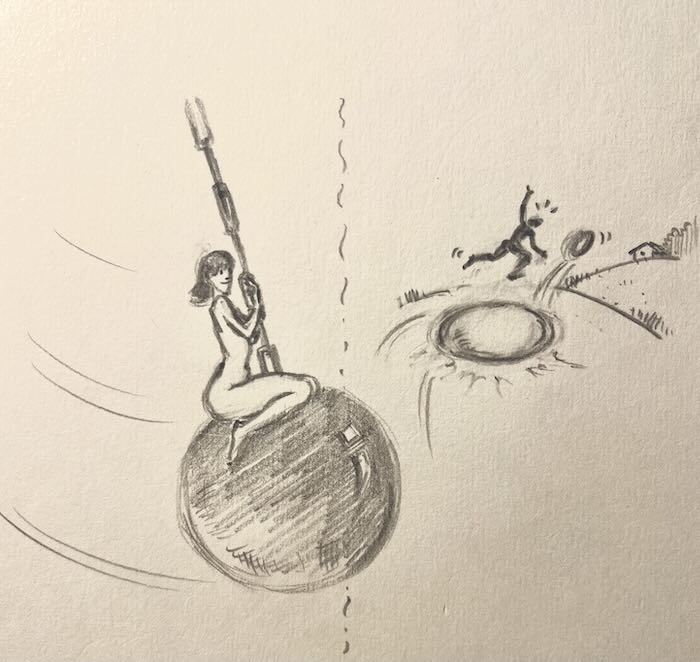
Verse Path and the Stepping Stones for the Metaverse
-----------------------------------------------------
TODO: Analogy to Pixar USD's Asset Path and to Houdini APEX KineFX Rigging Folder Path
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
Why Verse Is Not Necessarily Easy To Learn
==========================================
Given that one of the design goals Epic Games has for Verse is to make it
"learnable as a first language," Verse syntax overall has a clean and modern
look and feel, similar to Python. It appears closer to English and is less
cluttered with symbols. Additionally, Verse documentation is relatively
well-organized and informative. However, inexperienced users who set high hopes
based on these appearances may find themselves disillusioned when they start to
discover the language's deeper layers of complexity.
Arrays, Vectors, Lists
----------------------
Which API's Which
-----------------
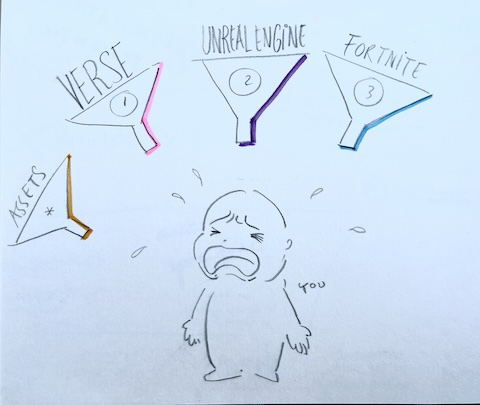
It's Okay to Have Specifiers, But Do You Need to Have That Many?
----------------------------------------------------------------
Verse doesn't merely adopt
[specifiers](https://dev.epicgames.com/documentation/en-us/uefn/specifiers-and-attributes-in-verse#effectspecifiers)
as C++ does; it seems to set a new benchmark with the sheer number of specifier
keywords it employs. This complexity might be off-putting to those who favor the
straightforwardness of Python. However, the beauty of Verse lies in its
foundation on "denotational semantics," a study that harmonizes modern language
features with the run-time safety Verse guarantees. Unlike Rust, which enforces
memory safety through a stringent borrow checker, Verse frees you from the
intricacies of low-level memory management. In the spirit of the "No free lunch"
adage in Machine Learning, the extensive use of specifiers is the trade-off for
achieving the robust safety and performance that Verse delivers.
*************************************************************************************************
* .-- converges
* +--- computes
* +--- varies
* +--- reads
* +--- writes
* +--- transacts
* +--- no_rollback .-- abstract
* EFFECT +--- decides +--- castable
* .--+--- suspends +--- concrete
* | '-- allocates +--- unique
* | CLASS +--- final
* +---------------------------+--- final_super
* | '-- epic_internal
* SPECIFIERS | .-- public
* IN VERSE ---+ +--- protected
* | ACCESS +--- private
* +---------+--- internal
* | '-- scoped
* | PERSISTENCE .-- persistable
* +------------------------------------+
* |
* | .-- native
* '--------------+
* IMPLEMENTATION '-- native_callable
*************************************************************************************************
!!! Tip
Although the list of specifiers may seem daunting, remember that you don't need
to understand or use all of them. Don't be discouraged by the specifiers; they
exist to enhance the language's capabilities and provide greater benefits.
Rapid Development
-----------------
TODO: The language is being rapidly developed, and thus still in flux. Watch out for
`deprecated` and `experimental` markers in the documentation.
TODO: E.g. `GetCreativeObjectsWithTag` has been superseded by `FindCreativeObjectsWithTag`
Use this example to highlight the importance of the fundamentals.
Structured and Unstructured Concurrency
---------------------------------------
TODO: Calling `spawn` multiple times for a same function means the resulting tasks can
all run in parallel (and the modifications are compounded) without us noticing.
Syntax Idiosyncracies
---------------------
!!!
Just like transitioning from one 3D software to another, such as Maya to
Blender, it's often the keyboard shortcuts that initially challenge users
rather than the design philosophy. Similarly, when learning a new
programming language, people often get bogged down by the syntax rather than
understanding the underlying concepts. Nevertheless, it's helpful to loudly
clarify some of the biggest syntax confusion now.
### The Tricky Question Mark
If you come from JavaScript and are familiar with the various uses of the
question mark operator, you'll have a head start. The table below illustrates
the different semantics of the question mark in Verse:
| 1. Optional Value | 2. Query Operator | 3. Named Argument |
|---------------------|----------------------|-----------------------------------|
| ? put before a type | ? put behind a value | ? put before a function parameter |
[Table [states]: Different meanings of the question mark.]
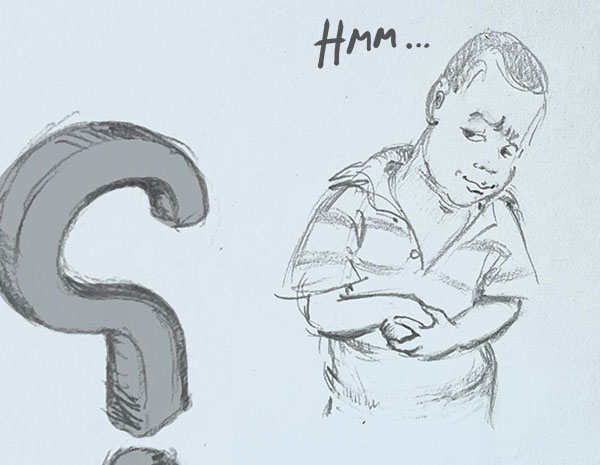
Let's look at an example for each of the three meanings:
- Meaning #1: `?` put before a type is used to denote an optional:
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ python
# We may or may not have a reference to this particle system component:
var MaybeParticle: ?particle_system_component = false
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
- Meaning #2: `?` put behind a value is used as query operator (the action is also known as unpacking or unwrapping):
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ python
var Is42TheAnswer: logic = true
# We query either an optional value or a logic value like this:
if (Is42TheAnswer?):
Print("Yes, it is.")
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
- Meaning #3: `?` put before a function parameter is used to denote named arguments:
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~ python
# This function takes a named argument OverTime:
FightTheGoodFight(?OverTime: float): fight_result = {}
# And then later in the code you call it by:
FightTheGoodFight(?OverTime:=AReallyLongTime)
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
### Square Brackets for Functions That Can Fail
It's easy to be deterred by small, strange things like the use of square
brackets in Verse. In most languages, "regular" functions are invoked with
parentheses `()`, but in Verse, **failable** functions are called with square
brackets `[]`. Some might find this syntax "abysmal," but it's all relative to
how we are used to manually checking return values. Instead, Verse leverages the
failure context to our advantage. Rust has a similar concept in [error
handling](https://doc.rust-lang.org/book/ch09-02-recoverable-errors-with-result.html#a-shortcut-for-propagating-errors-the--operator)
with its `Result` type; Verse just takes the notation a step further to make it
more explicit.
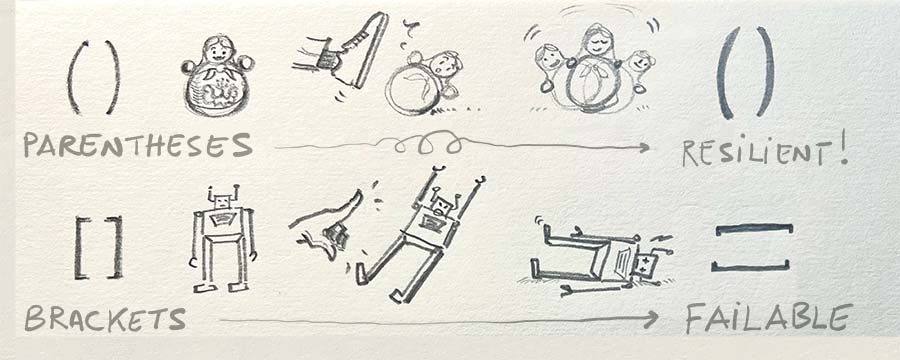
Remember that Verse is a brand-new language, created to free us from paradigms
that are popular but not necessarily beneficial. It's not just a functional
language; it's a functional **logic** language, designed to be different and
innovative. It doesn't have to follow the same conventions as other languages,
because it's not trying to be like them. It's trying to be better.
> "Why do what everyone else is doing, when everyone else is already doing it?"
> -- [Emil Ernerfeldt](https://github.com/emilk)
### Pascal case for variables, snake case for classes and types
This is not syntax, but it's a convention that may seem odd to some and take
some time to get used to.
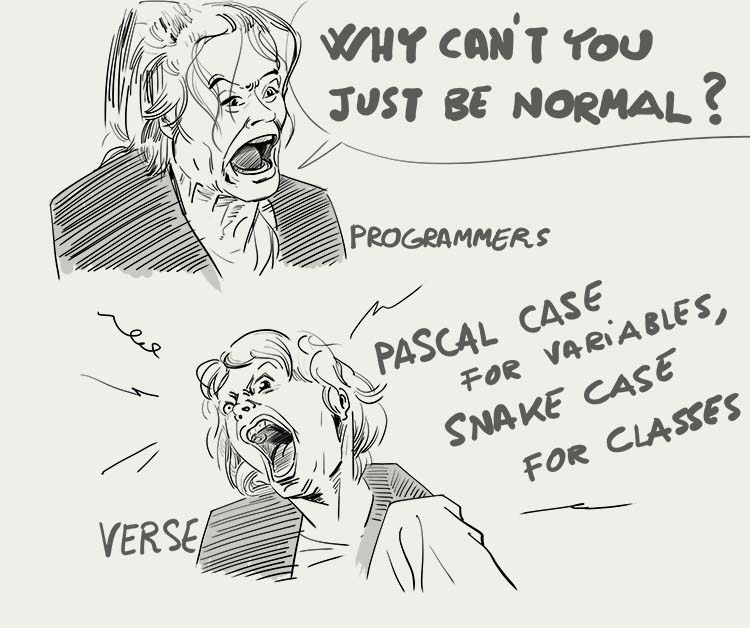
I find these conflicting conventions are just another manifestation of the old
debate of the universals and the particulars.
Component Lifetime
------------------
- Initialized
- AddedToScene
- BeginSimulation
(and then the most anticipated portion: the simulation loop)
- EndSimulation
- RemovingFromScene
- Uninitializing
TODO: elaborate on the distinction between device's `OnBegin` and component
`OnSimulate`.
Calling Lifetime
----------------
TODO: I.e. `rush` and `branch`.
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
A 90-Year Backstory
===================
Imperative Programming and the Dreaded Mutable State
----------------------------------------------------
In this section we shall talk briefly about the history of programming
languages, starting from the Turing Machine and the advent of imperative
programming languages.
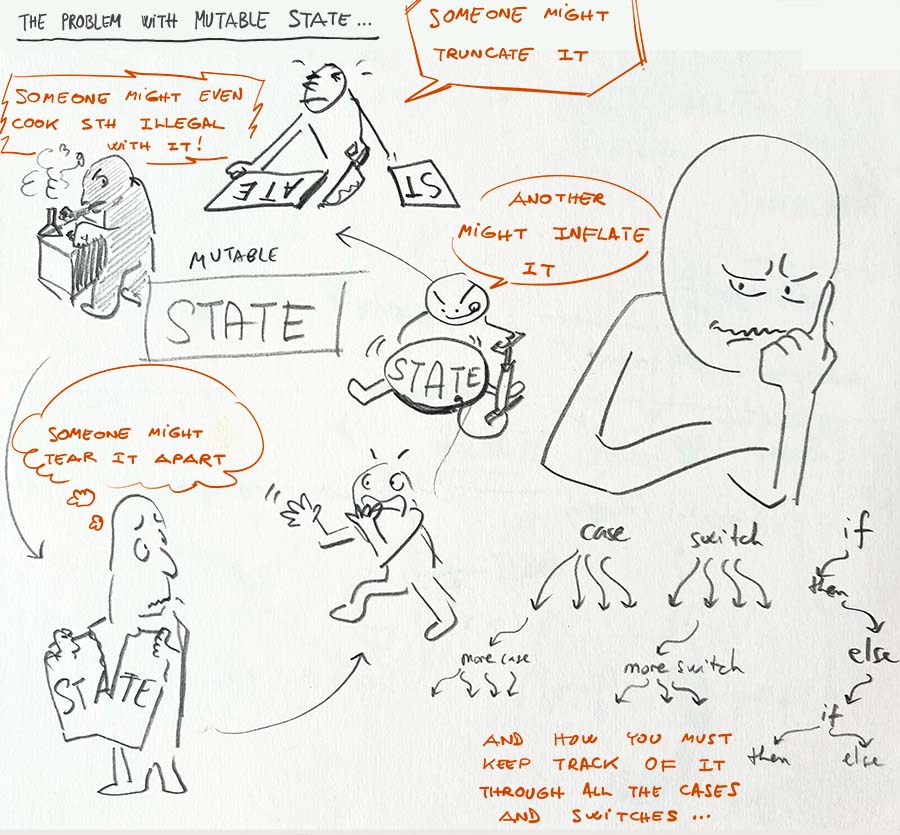
Church, Lambda Calculus, and Haskell
------------------------------------
!!!
Many mainstream programming languages today are imperative, and even though they
are "engineering marvels" and have been widely successful in terms of usefulness
and popularity, they share roots in the von Neumann architecture, which was
designed to execute instructions sequentially on mutable states. However, this
model has its [limitations](https://dl.acm.org/doi/10.1145/359576.359579), as it
can lead to bugs and make it difficult to reason about the behavior of a
program. In contrast, functional programming languages are declarative and based
on the principles of lambda calculus, developed by Alonzo Church. This approach
provides a way of expressing computations that has formal correctness, and also
is more elegant.
As a functional programming language, Verse offers a more robust and reliable
approach to software development. This is a bold move, considering the dominance
of imperative programming, which has persisted for over seventy years. Although
functional programming is often known for its steep learning curve, particularly
with concepts like Haskell's monad system, it has gained popularity in recent
years for its ability to address the limitations of imperative programming. One
positive quality of Verse is that it aims to eliminate the perceived academic
dryness often associated with functional programming, making it more accessible
and practical.
The Goodness Resulted from Tim Sweeney's Single-Mindedness
----------------------------------------------------------
> "When the limestone of imperative programming has worn away, [the granite of
> functional
> programming](https://www.microsoft.com/en-us/research/podcast/functional-programming-languages-pursuit-laziness-dr-simon-peyton-jones/)
> will be revealed underneath!"
> -- Simon Peyton Jones
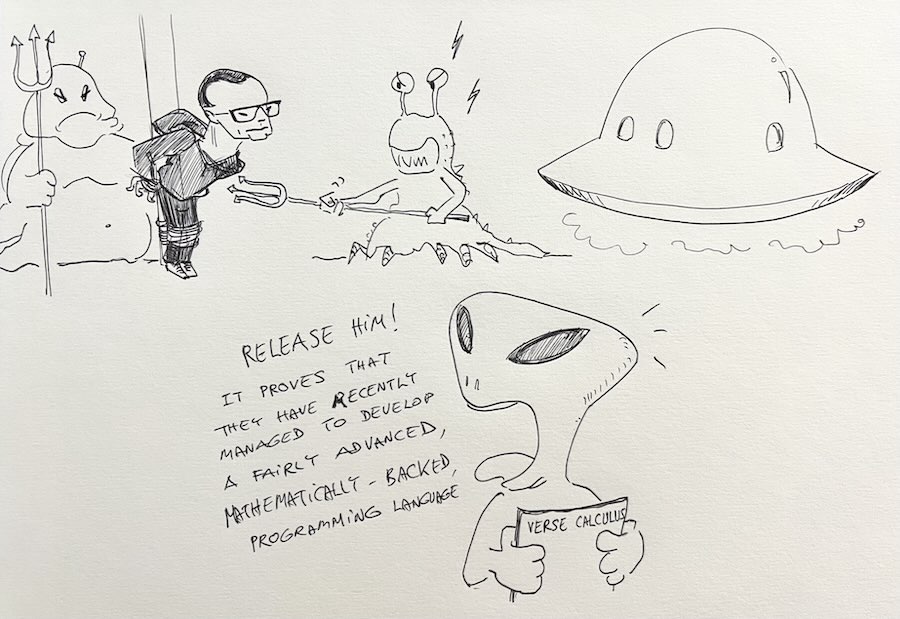
(#) Conclusion
(##) About the Author
Being a teacher and mentor since 2016 for over 800 Art and Tech Art students at
his founded school in various subjects ranging from Artistic Anatomy, Character
Rigging, Digital Sculpting, Python Scripting, Tool Development, and a life-long
learner himself, Hoan has a fascination with sharing knowledge and with
epistemology and pedagogy.
Specializing in character deformation as well as pipeline automation and
development, Hoan possesses keen eyes for artistic details and a love for
computer programming. The first programming language Hoan knew was Pascal in
1999; being gifted in drawing, nevertheless, he chose to go to Architecture
school in 2005, but then decided to shift to the animation industry when he
joined the 18-month Character Animation program at AnimationMentor in 2012 and
other Rigging courses online. His favorite authors are Christopher Alexander and
Isaac Asimov.
Starting with just modest scripts for VFX productions in 2016, Hoan gradually
taught himself Python in 2018 and later Rust in 2020 and became proficient with
them. Having worked at Virtuos and SPARX* for six years and shipped multiple
games and products, he was trusted the most with R&D, pioneering techniques, and
training teams. He also has a passion for developing interactive mobile apps
that aid student learning.
Contrary to what most people think about technical persons, Hoan also loves
acting and has a sense of humor. During high school, he used to write plays and
sketches, then form groups from his circle of friends and direct them. He
usually carried out the set dressing, the music, and the staging himself in
those comedies. He stumbled upon two books on Scheme in 2010, not being able to
fully grasp the content at the time, but he kept them carefully until now.
Having completed the Unreal Fellowship 2024 — the first of its kind about Games
— and published his resulting FOSSIL SKATER game on Epic Games Store and Steam,
Hoan learned a lot about making games in Unreal with Blueprints in a shorter
amount of time than he ever anticipated before joining the program. His game is
a madcap racing game where you ride dinosaurs who skate on "car-shoes" towards
erupting volcanoes.
Born in a rural mountainous area in post-war Vietnam, Hoan appreciates the
learning opportunities he had when studying abroad in the USA in 2008, and
continues to value them after migrating to the USA 15 years later. First hearing
about UEFN and Verse during the Fellowship, he then went to Unreal Fest Seattle
2024 to connect with like-minded fellows across the globe and also to see how
Epic Games is pushing the Verse programming language.
He has tried to learn Verse and Haskell as much as he can since November 2024,
and now wants to talk to people about Verse's awesomeness.
(##) Feedback
If you have any feedback or suggestions, please feel free to reach out to me at
info (at) mushogenshin (dot) com.